C++ lambda syntaxWhat is the difference between syntax and semantics of programming languages?What are the differences between a pointer variable and a reference variable in C++?What is the difference between a 'closure' and a 'lambda'?How can I profile C++ code running on Linux?The Definitive C++ Book Guide and ListWhy are Python lambdas useful?What is the effect of extern “C” in C++?Distinct() with lambda?What is the “-->” operator in C++?What is a lambda expression in C++11?Why is reading lines from stdin much slower in C++ than Python?
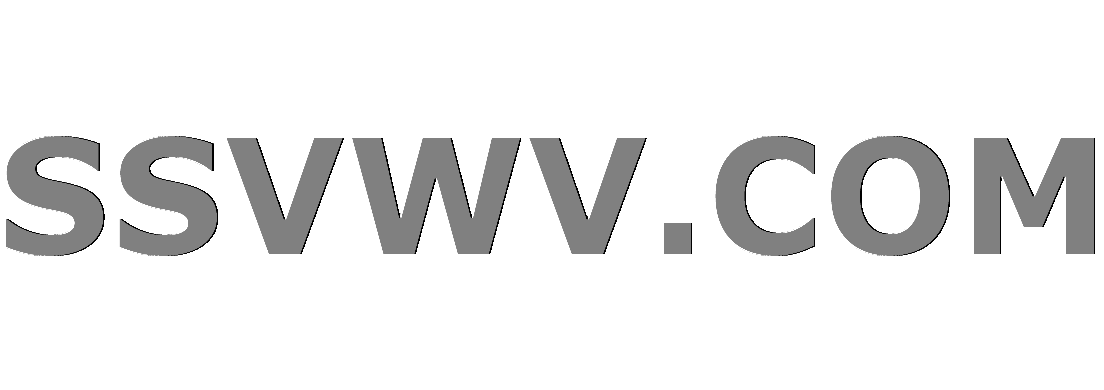
Multi tool use
Friend wants my recommendation but I don't want to give it to him
Sort with assumptions
How to split IPA spelling into syllables
Not hide and seek
Are hand made posters acceptable in Academia?
"Oh no!" in Latin
What do the positive and negative (+/-) transmit and receive pins mean on Ethernet cables?
Did I make a mistake by ccing email to boss to others?
Center page as a whole without centering each element individually
How to preserve electronics (computers, ipads, phones) for hundreds of years?
Relations between homogeneous polynomials
Trouble reading roman numeral notation with flats
categorizing a variable turns it from insignificant to significant
Weird lines in Microsoft Word
Why is implicit conversion not ambiguous for non-primitive types?
PTIJ: Which Dr. Seuss books should one obtain?
Should a narrator ever describe things based on a character's view instead of facts?
Why does the frost depth increase when the surface temperature warms up?
Can a Knock spell open the door to Mordenkainen's Magnificent Mansion?
Can you describe someone as luxurious? As in someone who likes luxurious things?
Travelling in US for more than 90 days
Checking @@ROWCOUNT failing
New Order #2: Turn My Way
Air travel with refrigerated insulin
C++ lambda syntax
What is the difference between syntax and semantics of programming languages?What are the differences between a pointer variable and a reference variable in C++?What is the difference between a 'closure' and a 'lambda'?How can I profile C++ code running on Linux?The Definitive C++ Book Guide and ListWhy are Python lambdas useful?What is the effect of extern “C” in C++?Distinct() with lambda?What is the “-->” operator in C++?What is a lambda expression in C++11?Why is reading lines from stdin much slower in C++ than Python?
I have a function that searches a vector of iterators and returns the iterator if its names matches a string passed as an argument.
koalaGraph::PVertex lookUpByName(std::string Name, std::vector<koalaGraph::PVertex>& Vertices)
for (size_t i = 0; i < Vertices.size(); i++)
if(Vertices[i]->info.name == Name)
return Vertices[i];
My question is how can I implement this as a lamda, I'm using std:find_if
?
I'm trying this:
std::vector<koalaGraph::PVertex> V
std:string Name;
std::find_if(V.begin(), V.end(), [&Name]() return Name == V->info.name;)
But it says that V
an enclosing-function local variable cannot be referenced in a lambda body unless it is in the capture list
c++ lambda
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I have a function that searches a vector of iterators and returns the iterator if its names matches a string passed as an argument.
koalaGraph::PVertex lookUpByName(std::string Name, std::vector<koalaGraph::PVertex>& Vertices)
for (size_t i = 0; i < Vertices.size(); i++)
if(Vertices[i]->info.name == Name)
return Vertices[i];
My question is how can I implement this as a lamda, I'm using std:find_if
?
I'm trying this:
std::vector<koalaGraph::PVertex> V
std:string Name;
std::find_if(V.begin(), V.end(), [&Name]() return Name == V->info.name;)
But it says that V
an enclosing-function local variable cannot be referenced in a lambda body unless it is in the capture list
c++ lambda
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Noreturn
if nothing found?
– Deduplicator
4 hours ago
add a comment |
I have a function that searches a vector of iterators and returns the iterator if its names matches a string passed as an argument.
koalaGraph::PVertex lookUpByName(std::string Name, std::vector<koalaGraph::PVertex>& Vertices)
for (size_t i = 0; i < Vertices.size(); i++)
if(Vertices[i]->info.name == Name)
return Vertices[i];
My question is how can I implement this as a lamda, I'm using std:find_if
?
I'm trying this:
std::vector<koalaGraph::PVertex> V
std:string Name;
std::find_if(V.begin(), V.end(), [&Name]() return Name == V->info.name;)
But it says that V
an enclosing-function local variable cannot be referenced in a lambda body unless it is in the capture list
c++ lambda
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I have a function that searches a vector of iterators and returns the iterator if its names matches a string passed as an argument.
koalaGraph::PVertex lookUpByName(std::string Name, std::vector<koalaGraph::PVertex>& Vertices)
for (size_t i = 0; i < Vertices.size(); i++)
if(Vertices[i]->info.name == Name)
return Vertices[i];
My question is how can I implement this as a lamda, I'm using std:find_if
?
I'm trying this:
std::vector<koalaGraph::PVertex> V
std:string Name;
std::find_if(V.begin(), V.end(), [&Name]() return Name == V->info.name;)
But it says that V
an enclosing-function local variable cannot be referenced in a lambda body unless it is in the capture list
c++ lambda
c++ lambda
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 11 hours ago
Quentin
46.5k589146
46.5k589146
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 11 hours ago


black sheepblack sheep
521
521
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
black sheep is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Noreturn
if nothing found?
– Deduplicator
4 hours ago
add a comment |
1
Noreturn
if nothing found?
– Deduplicator
4 hours ago
1
1
No
return
if nothing found?– Deduplicator
4 hours ago
No
return
if nothing found?– Deduplicator
4 hours ago
add a comment |
5 Answers
5
active
oldest
votes
find_if
is going to pass the elements of the vector into your lambda. That means you need
std::find_if(V.begin(), V.end(), [&Name](auto const& V) return Name == V->info.name;)
so that the V
in the lambda body is the element of the vector, not the vector itself.
Ideally you'd give it a different name than V
so you keep the vector and local variables separate like
std::find_if(V.begin(), V.end(), [&Name](auto const& element) return Name == elememt->info.name;)
So now it is clear you are working on a element of the vector, instead of the vector itself.
3
It would be better not to use the same nameV
for two different things (the vector and the iterator). Thus,std::find_if(V.begin(), V.end(), [&Name](auto const& it) return Name == it->info.name;)
would be better.
– Handy999
6 hours ago
@Handy999it
is also misleading though, since it's not an iterator. Maybevalue
,element
or whatever else :)
– Rakete1111
6 hours ago
@Handy999 You're right about giving it a better name, I just wanted to keep it asV
as it makes my last sentence very succinct.
– NathanOliver
6 hours ago
add a comment |
First, V->info.name
is ill formed, inside or outside of the lambda.
The function object sent to the algoritm std::find_if
must be a unary function. It must take the current element to check as a parameter.
auto found = std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const& item_to_check)
return Name == item_to_check->info.name;
);
The type of found
is an iterator to the element that has been found. If none is found, then it returns V.end()
If you use C++14 or better, you can even use generic lambdas:
auto found = std::find_if(
V.begin(), V.end(),
[&Name](auto const& item_to_check)
return Name == item_to_check->info.name;
);
V->info.name
is valid syntax. It just won't typecheck since vectors don't overload->
.
– Silvio Mayolo
11 hours ago
2
It just won't typecheck since vectors don't overload ->
so... it won't compile? It's an invalid syntax to use->
on object of types that don't overloadoperator->
– Guillaume Racicot
11 hours ago
Not all compile errors are syntax errors. See syntax vs. semantics.
– Silvio Mayolo
11 hours ago
1
@SilvioMayolo The answer you linked me says that semantics is the meaning of the program, usually in it's runtime behaviour. The code I quoted is simply ill formed, there is no semantic yet. Or maybe I don't understand the concept correctly
– Guillaume Racicot
11 hours ago
add a comment |
std::find_if
's predicate will receive a reference to each element of the range in turn. You need:
std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const &v) return Name == v->info.name;
);
add a comment |
Get V
as parameter to the lambda.
std::find_if(V.begin(), V.end(), [&Name](type& V) {return Name == V->info.name;)
add a comment |
Use const auto &
to access the individual elements from your vector in the lambda expression. Since the vector is an lvalue, auto will be deduced to const vector<PVertex> &
. Then, you can use std::distance
to find the element location of the object in the vector.
struct PVertex
std::string name;
;
int main()
std::vector<PVertex> V = "foo","bar","cat","dog";
std::string Name = "cat";
auto found = std::find_if(std::begin(V), std::end(V), [&Name](const auto &v)return (Name == v.name););
std::cout<< "found at: V["<<std::distance(std::begin(V),found)<<"]" <<std::endl;
Result is:
found at: V[2]
Example: https://rextester.com/IYNA58046
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
black sheep is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55243276%2fc-lambda-syntax%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
find_if
is going to pass the elements of the vector into your lambda. That means you need
std::find_if(V.begin(), V.end(), [&Name](auto const& V) return Name == V->info.name;)
so that the V
in the lambda body is the element of the vector, not the vector itself.
Ideally you'd give it a different name than V
so you keep the vector and local variables separate like
std::find_if(V.begin(), V.end(), [&Name](auto const& element) return Name == elememt->info.name;)
So now it is clear you are working on a element of the vector, instead of the vector itself.
3
It would be better not to use the same nameV
for two different things (the vector and the iterator). Thus,std::find_if(V.begin(), V.end(), [&Name](auto const& it) return Name == it->info.name;)
would be better.
– Handy999
6 hours ago
@Handy999it
is also misleading though, since it's not an iterator. Maybevalue
,element
or whatever else :)
– Rakete1111
6 hours ago
@Handy999 You're right about giving it a better name, I just wanted to keep it asV
as it makes my last sentence very succinct.
– NathanOliver
6 hours ago
add a comment |
find_if
is going to pass the elements of the vector into your lambda. That means you need
std::find_if(V.begin(), V.end(), [&Name](auto const& V) return Name == V->info.name;)
so that the V
in the lambda body is the element of the vector, not the vector itself.
Ideally you'd give it a different name than V
so you keep the vector and local variables separate like
std::find_if(V.begin(), V.end(), [&Name](auto const& element) return Name == elememt->info.name;)
So now it is clear you are working on a element of the vector, instead of the vector itself.
3
It would be better not to use the same nameV
for two different things (the vector and the iterator). Thus,std::find_if(V.begin(), V.end(), [&Name](auto const& it) return Name == it->info.name;)
would be better.
– Handy999
6 hours ago
@Handy999it
is also misleading though, since it's not an iterator. Maybevalue
,element
or whatever else :)
– Rakete1111
6 hours ago
@Handy999 You're right about giving it a better name, I just wanted to keep it asV
as it makes my last sentence very succinct.
– NathanOliver
6 hours ago
add a comment |
find_if
is going to pass the elements of the vector into your lambda. That means you need
std::find_if(V.begin(), V.end(), [&Name](auto const& V) return Name == V->info.name;)
so that the V
in the lambda body is the element of the vector, not the vector itself.
Ideally you'd give it a different name than V
so you keep the vector and local variables separate like
std::find_if(V.begin(), V.end(), [&Name](auto const& element) return Name == elememt->info.name;)
So now it is clear you are working on a element of the vector, instead of the vector itself.
find_if
is going to pass the elements of the vector into your lambda. That means you need
std::find_if(V.begin(), V.end(), [&Name](auto const& V) return Name == V->info.name;)
so that the V
in the lambda body is the element of the vector, not the vector itself.
Ideally you'd give it a different name than V
so you keep the vector and local variables separate like
std::find_if(V.begin(), V.end(), [&Name](auto const& element) return Name == elememt->info.name;)
So now it is clear you are working on a element of the vector, instead of the vector itself.
edited 4 hours ago
answered 11 hours ago


NathanOliverNathanOliver
95.6k16135208
95.6k16135208
3
It would be better not to use the same nameV
for two different things (the vector and the iterator). Thus,std::find_if(V.begin(), V.end(), [&Name](auto const& it) return Name == it->info.name;)
would be better.
– Handy999
6 hours ago
@Handy999it
is also misleading though, since it's not an iterator. Maybevalue
,element
or whatever else :)
– Rakete1111
6 hours ago
@Handy999 You're right about giving it a better name, I just wanted to keep it asV
as it makes my last sentence very succinct.
– NathanOliver
6 hours ago
add a comment |
3
It would be better not to use the same nameV
for two different things (the vector and the iterator). Thus,std::find_if(V.begin(), V.end(), [&Name](auto const& it) return Name == it->info.name;)
would be better.
– Handy999
6 hours ago
@Handy999it
is also misleading though, since it's not an iterator. Maybevalue
,element
or whatever else :)
– Rakete1111
6 hours ago
@Handy999 You're right about giving it a better name, I just wanted to keep it asV
as it makes my last sentence very succinct.
– NathanOliver
6 hours ago
3
3
It would be better not to use the same name
V
for two different things (the vector and the iterator). Thus, std::find_if(V.begin(), V.end(), [&Name](auto const& it) return Name == it->info.name;)
would be better.– Handy999
6 hours ago
It would be better not to use the same name
V
for two different things (the vector and the iterator). Thus, std::find_if(V.begin(), V.end(), [&Name](auto const& it) return Name == it->info.name;)
would be better.– Handy999
6 hours ago
@Handy999
it
is also misleading though, since it's not an iterator. Maybe value
, element
or whatever else :)– Rakete1111
6 hours ago
@Handy999
it
is also misleading though, since it's not an iterator. Maybe value
, element
or whatever else :)– Rakete1111
6 hours ago
@Handy999 You're right about giving it a better name, I just wanted to keep it as
V
as it makes my last sentence very succinct.– NathanOliver
6 hours ago
@Handy999 You're right about giving it a better name, I just wanted to keep it as
V
as it makes my last sentence very succinct.– NathanOliver
6 hours ago
add a comment |
First, V->info.name
is ill formed, inside or outside of the lambda.
The function object sent to the algoritm std::find_if
must be a unary function. It must take the current element to check as a parameter.
auto found = std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const& item_to_check)
return Name == item_to_check->info.name;
);
The type of found
is an iterator to the element that has been found. If none is found, then it returns V.end()
If you use C++14 or better, you can even use generic lambdas:
auto found = std::find_if(
V.begin(), V.end(),
[&Name](auto const& item_to_check)
return Name == item_to_check->info.name;
);
V->info.name
is valid syntax. It just won't typecheck since vectors don't overload->
.
– Silvio Mayolo
11 hours ago
2
It just won't typecheck since vectors don't overload ->
so... it won't compile? It's an invalid syntax to use->
on object of types that don't overloadoperator->
– Guillaume Racicot
11 hours ago
Not all compile errors are syntax errors. See syntax vs. semantics.
– Silvio Mayolo
11 hours ago
1
@SilvioMayolo The answer you linked me says that semantics is the meaning of the program, usually in it's runtime behaviour. The code I quoted is simply ill formed, there is no semantic yet. Or maybe I don't understand the concept correctly
– Guillaume Racicot
11 hours ago
add a comment |
First, V->info.name
is ill formed, inside or outside of the lambda.
The function object sent to the algoritm std::find_if
must be a unary function. It must take the current element to check as a parameter.
auto found = std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const& item_to_check)
return Name == item_to_check->info.name;
);
The type of found
is an iterator to the element that has been found. If none is found, then it returns V.end()
If you use C++14 or better, you can even use generic lambdas:
auto found = std::find_if(
V.begin(), V.end(),
[&Name](auto const& item_to_check)
return Name == item_to_check->info.name;
);
V->info.name
is valid syntax. It just won't typecheck since vectors don't overload->
.
– Silvio Mayolo
11 hours ago
2
It just won't typecheck since vectors don't overload ->
so... it won't compile? It's an invalid syntax to use->
on object of types that don't overloadoperator->
– Guillaume Racicot
11 hours ago
Not all compile errors are syntax errors. See syntax vs. semantics.
– Silvio Mayolo
11 hours ago
1
@SilvioMayolo The answer you linked me says that semantics is the meaning of the program, usually in it's runtime behaviour. The code I quoted is simply ill formed, there is no semantic yet. Or maybe I don't understand the concept correctly
– Guillaume Racicot
11 hours ago
add a comment |
First, V->info.name
is ill formed, inside or outside of the lambda.
The function object sent to the algoritm std::find_if
must be a unary function. It must take the current element to check as a parameter.
auto found = std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const& item_to_check)
return Name == item_to_check->info.name;
);
The type of found
is an iterator to the element that has been found. If none is found, then it returns V.end()
If you use C++14 or better, you can even use generic lambdas:
auto found = std::find_if(
V.begin(), V.end(),
[&Name](auto const& item_to_check)
return Name == item_to_check->info.name;
);
First, V->info.name
is ill formed, inside or outside of the lambda.
The function object sent to the algoritm std::find_if
must be a unary function. It must take the current element to check as a parameter.
auto found = std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const& item_to_check)
return Name == item_to_check->info.name;
);
The type of found
is an iterator to the element that has been found. If none is found, then it returns V.end()
If you use C++14 or better, you can even use generic lambdas:
auto found = std::find_if(
V.begin(), V.end(),
[&Name](auto const& item_to_check)
return Name == item_to_check->info.name;
);
edited 11 hours ago
answered 11 hours ago
Guillaume RacicotGuillaume Racicot
15.3k53568
15.3k53568
V->info.name
is valid syntax. It just won't typecheck since vectors don't overload->
.
– Silvio Mayolo
11 hours ago
2
It just won't typecheck since vectors don't overload ->
so... it won't compile? It's an invalid syntax to use->
on object of types that don't overloadoperator->
– Guillaume Racicot
11 hours ago
Not all compile errors are syntax errors. See syntax vs. semantics.
– Silvio Mayolo
11 hours ago
1
@SilvioMayolo The answer you linked me says that semantics is the meaning of the program, usually in it's runtime behaviour. The code I quoted is simply ill formed, there is no semantic yet. Or maybe I don't understand the concept correctly
– Guillaume Racicot
11 hours ago
add a comment |
V->info.name
is valid syntax. It just won't typecheck since vectors don't overload->
.
– Silvio Mayolo
11 hours ago
2
It just won't typecheck since vectors don't overload ->
so... it won't compile? It's an invalid syntax to use->
on object of types that don't overloadoperator->
– Guillaume Racicot
11 hours ago
Not all compile errors are syntax errors. See syntax vs. semantics.
– Silvio Mayolo
11 hours ago
1
@SilvioMayolo The answer you linked me says that semantics is the meaning of the program, usually in it's runtime behaviour. The code I quoted is simply ill formed, there is no semantic yet. Or maybe I don't understand the concept correctly
– Guillaume Racicot
11 hours ago
V->info.name
is valid syntax. It just won't typecheck since vectors don't overload ->
.– Silvio Mayolo
11 hours ago
V->info.name
is valid syntax. It just won't typecheck since vectors don't overload ->
.– Silvio Mayolo
11 hours ago
2
2
It just won't typecheck since vectors don't overload ->
so... it won't compile? It's an invalid syntax to use ->
on object of types that don't overload operator->
– Guillaume Racicot
11 hours ago
It just won't typecheck since vectors don't overload ->
so... it won't compile? It's an invalid syntax to use ->
on object of types that don't overload operator->
– Guillaume Racicot
11 hours ago
Not all compile errors are syntax errors. See syntax vs. semantics.
– Silvio Mayolo
11 hours ago
Not all compile errors are syntax errors. See syntax vs. semantics.
– Silvio Mayolo
11 hours ago
1
1
@SilvioMayolo The answer you linked me says that semantics is the meaning of the program, usually in it's runtime behaviour. The code I quoted is simply ill formed, there is no semantic yet. Or maybe I don't understand the concept correctly
– Guillaume Racicot
11 hours ago
@SilvioMayolo The answer you linked me says that semantics is the meaning of the program, usually in it's runtime behaviour. The code I quoted is simply ill formed, there is no semantic yet. Or maybe I don't understand the concept correctly
– Guillaume Racicot
11 hours ago
add a comment |
std::find_if
's predicate will receive a reference to each element of the range in turn. You need:
std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const &v) return Name == v->info.name;
);
add a comment |
std::find_if
's predicate will receive a reference to each element of the range in turn. You need:
std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const &v) return Name == v->info.name;
);
add a comment |
std::find_if
's predicate will receive a reference to each element of the range in turn. You need:
std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const &v) return Name == v->info.name;
);
std::find_if
's predicate will receive a reference to each element of the range in turn. You need:
std::find_if(
V.begin(), V.end(),
[&Name](koalaGraph::PVertex const &v) return Name == v->info.name;
);
answered 11 hours ago
QuentinQuentin
46.5k589146
46.5k589146
add a comment |
add a comment |
Get V
as parameter to the lambda.
std::find_if(V.begin(), V.end(), [&Name](type& V) {return Name == V->info.name;)
add a comment |
Get V
as parameter to the lambda.
std::find_if(V.begin(), V.end(), [&Name](type& V) {return Name == V->info.name;)
add a comment |
Get V
as parameter to the lambda.
std::find_if(V.begin(), V.end(), [&Name](type& V) {return Name == V->info.name;)
Get V
as parameter to the lambda.
std::find_if(V.begin(), V.end(), [&Name](type& V) {return Name == V->info.name;)
answered 11 hours ago
Petar VelevPetar Velev
1,671619
1,671619
add a comment |
add a comment |
Use const auto &
to access the individual elements from your vector in the lambda expression. Since the vector is an lvalue, auto will be deduced to const vector<PVertex> &
. Then, you can use std::distance
to find the element location of the object in the vector.
struct PVertex
std::string name;
;
int main()
std::vector<PVertex> V = "foo","bar","cat","dog";
std::string Name = "cat";
auto found = std::find_if(std::begin(V), std::end(V), [&Name](const auto &v)return (Name == v.name););
std::cout<< "found at: V["<<std::distance(std::begin(V),found)<<"]" <<std::endl;
Result is:
found at: V[2]
Example: https://rextester.com/IYNA58046
add a comment |
Use const auto &
to access the individual elements from your vector in the lambda expression. Since the vector is an lvalue, auto will be deduced to const vector<PVertex> &
. Then, you can use std::distance
to find the element location of the object in the vector.
struct PVertex
std::string name;
;
int main()
std::vector<PVertex> V = "foo","bar","cat","dog";
std::string Name = "cat";
auto found = std::find_if(std::begin(V), std::end(V), [&Name](const auto &v)return (Name == v.name););
std::cout<< "found at: V["<<std::distance(std::begin(V),found)<<"]" <<std::endl;
Result is:
found at: V[2]
Example: https://rextester.com/IYNA58046
add a comment |
Use const auto &
to access the individual elements from your vector in the lambda expression. Since the vector is an lvalue, auto will be deduced to const vector<PVertex> &
. Then, you can use std::distance
to find the element location of the object in the vector.
struct PVertex
std::string name;
;
int main()
std::vector<PVertex> V = "foo","bar","cat","dog";
std::string Name = "cat";
auto found = std::find_if(std::begin(V), std::end(V), [&Name](const auto &v)return (Name == v.name););
std::cout<< "found at: V["<<std::distance(std::begin(V),found)<<"]" <<std::endl;
Result is:
found at: V[2]
Example: https://rextester.com/IYNA58046
Use const auto &
to access the individual elements from your vector in the lambda expression. Since the vector is an lvalue, auto will be deduced to const vector<PVertex> &
. Then, you can use std::distance
to find the element location of the object in the vector.
struct PVertex
std::string name;
;
int main()
std::vector<PVertex> V = "foo","bar","cat","dog";
std::string Name = "cat";
auto found = std::find_if(std::begin(V), std::end(V), [&Name](const auto &v)return (Name == v.name););
std::cout<< "found at: V["<<std::distance(std::begin(V),found)<<"]" <<std::endl;
Result is:
found at: V[2]
Example: https://rextester.com/IYNA58046
answered 11 hours ago


Constantinos GlynosConstantinos Glynos
1,581820
1,581820
add a comment |
add a comment |
black sheep is a new contributor. Be nice, and check out our Code of Conduct.
black sheep is a new contributor. Be nice, and check out our Code of Conduct.
black sheep is a new contributor. Be nice, and check out our Code of Conduct.
black sheep is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55243276%2fc-lambda-syntax%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PM0HK7,DFMuBw,CTZJEAz,YwVY8si4xBvNzLc 9prg7xpDQ3qL 0JJVfvoCm0BCNkD8J KCUK0zl QSXF,wt1C57qGlo2eBY,YTAUfP
1
No
return
if nothing found?– Deduplicator
4 hours ago